Software documentation is an essential part of software development, providing information about the code and how it works. It is a critical part of the development process that helps developers understand and maintain the code, as well as make it easier for others to contribute to the project. Documentation is especially important in dynamic and rapidly evolving languages like JavaScript.
JavaScript is a popular language for building web applications, and its popularity has only increased with the rise of frameworks like React and Angular. JavaScript developers are expected to write clean, readable, and well-documented code that can be easily understood by other developers. Documentation can take many forms, including inline comments, README files, and API documentation.
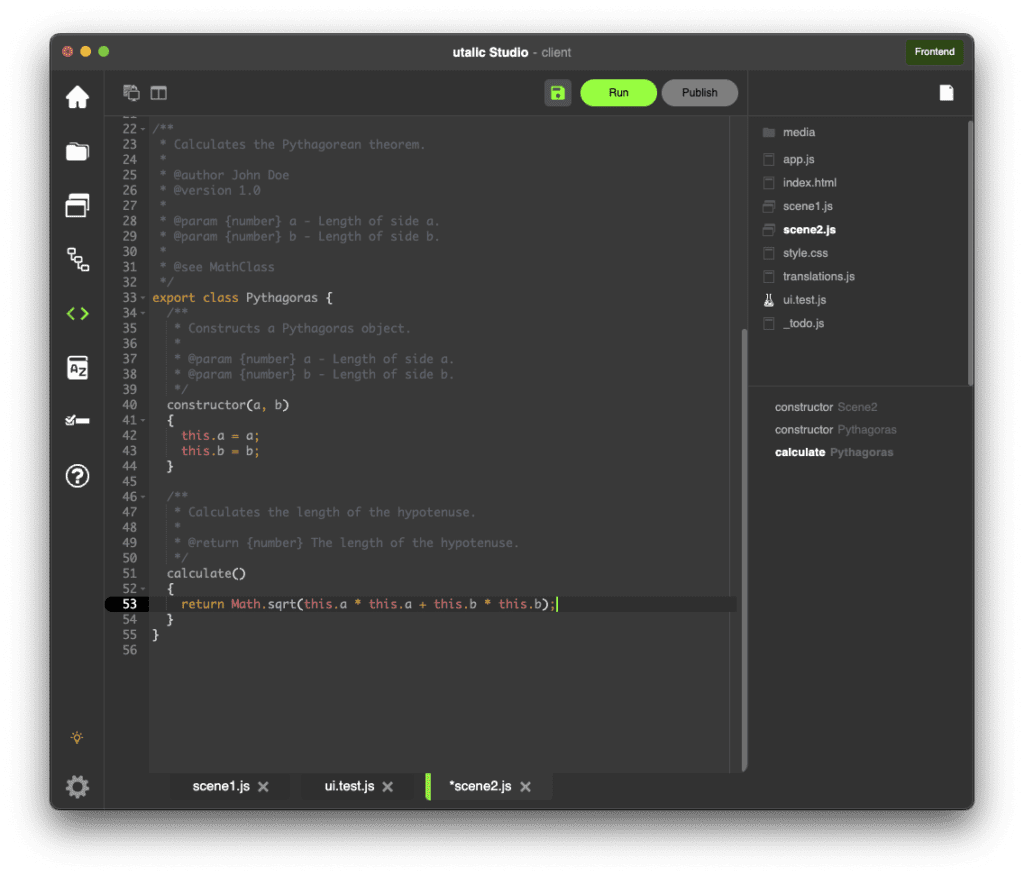
Inline Comments in JavaScript
Inline comments are an essential part of documenting your code. They allow you to explain what a specific part of your code does and why it’s important. In JavaScript, inline comments are created by starting a line with two slashes (//). Here’s an example of a commented method in JavaScript:
// This function adds two numbers together
function addNumbers(a, b) {
// Calculate the result
let result = a + b;
// Return the result
return result;
}
In this example, the inline comments explain what the function does and what each line of code is doing. These comments help other developers understand the code, especially if they are unfamiliar with the project. They also help you remember why you wrote the code a certain way if you revisit it later.
Documenting Variables and Functions
In addition to inline comments, it’s important to document your variables and functions. This documentation should include information about what the variable or function does, what it takes as inputs, and what it returns as output. Here’s an example of a well-documented function in JavaScript:
/**
* Adds two numbers together and returns the result
*
* @param {Number} a - The first number to add
* @param {Number} b - The second number to add
*
* @return {Number} The result of adding the two numbers together
*/
function addNumbers(a, b) {
let result = a + b;
return result;
}
In this example, the documentation uses the JavaScript doc-block syntax, which is a special type of comment that starts with /** and ends with */. This syntax allows you to create detailed documentation that can be automatically processed by tools like JSDoc, which generates API documentation from your code.
Conclusion
Software documentation is a critical part of the software development process, especially in rapidly evolving languages like JavaScript. Documentation helps developers understand the code, makes it easier for others to contribute, and helps you maintain the code over time. Inline comments, variable and function documentation, and API documentation are all important parts of the documentation process. By taking the time to document your code, you can ensure that your projects are maintainable and that your code is easy to understand for years to come.